|
| 1 | +# 2130. Maximum Twin Sum of a Linked List |
| 2 | + |
| 3 | +- Difficulty: Medium. |
| 4 | +- Related Topics: Linked List, Two Pointers, Stack. |
| 5 | +- Similar Questions: Reverse Linked List, Palindrome Linked List, Middle of the Linked List. |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +In a linked list of size `n`, where `n` is **even**, the `ith` node (**0-indexed**) of the linked list is known as the **twin** of the `(n-1-i)th` node, if `0 <= i <= (n / 2) - 1`. |
| 10 | + |
| 11 | + |
| 12 | + |
| 13 | +- For example, if `n = 4`, then node `0` is the twin of node `3`, and node `1` is the twin of node `2`. These are the only nodes with twins for `n = 4`. |
| 14 | + |
| 15 | + |
| 16 | +The **twin sum **is defined as the sum of a node and its twin. |
| 17 | + |
| 18 | +Given the `head` of a linked list with even length, return **the **maximum twin sum** of the linked list**. |
| 19 | + |
| 20 | + |
| 21 | +Example 1: |
| 22 | + |
| 23 | +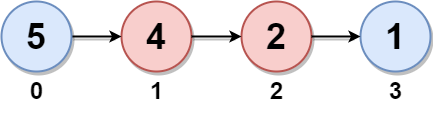 |
| 24 | + |
| 25 | +``` |
| 26 | +Input: head = [5,4,2,1] |
| 27 | +Output: 6 |
| 28 | +Explanation: |
| 29 | +Nodes 0 and 1 are the twins of nodes 3 and 2, respectively. All have twin sum = 6. |
| 30 | +There are no other nodes with twins in the linked list. |
| 31 | +Thus, the maximum twin sum of the linked list is 6. |
| 32 | +``` |
| 33 | + |
| 34 | +Example 2: |
| 35 | + |
| 36 | +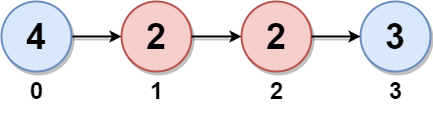 |
| 37 | + |
| 38 | +``` |
| 39 | +Input: head = [4,2,2,3] |
| 40 | +Output: 7 |
| 41 | +Explanation: |
| 42 | +The nodes with twins present in this linked list are: |
| 43 | +- Node 0 is the twin of node 3 having a twin sum of 4 + 3 = 7. |
| 44 | +- Node 1 is the twin of node 2 having a twin sum of 2 + 2 = 4. |
| 45 | +Thus, the maximum twin sum of the linked list is max(7, 4) = 7. |
| 46 | +``` |
| 47 | + |
| 48 | +Example 3: |
| 49 | + |
| 50 | +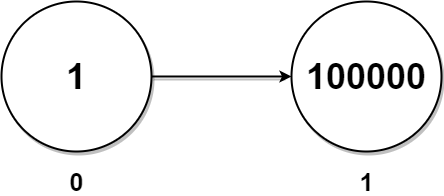 |
| 51 | + |
| 52 | +``` |
| 53 | +Input: head = [1,100000] |
| 54 | +Output: 100001 |
| 55 | +Explanation: |
| 56 | +There is only one node with a twin in the linked list having twin sum of 1 + 100000 = 100001. |
| 57 | +``` |
| 58 | + |
| 59 | + |
| 60 | +**Constraints:** |
| 61 | + |
| 62 | + |
| 63 | + |
| 64 | +- The number of nodes in the list is an **even** integer in the range `[2, 105]`. |
| 65 | + |
| 66 | +- `1 <= Node.val <= 105` |
| 67 | + |
| 68 | + |
| 69 | + |
| 70 | +## Solution |
| 71 | + |
| 72 | +```javascript |
| 73 | +/** |
| 74 | + * Definition for singly-linked list. |
| 75 | + * function ListNode(val, next) { |
| 76 | + * this.val = (val===undefined ? 0 : val) |
| 77 | + * this.next = (next===undefined ? null : next) |
| 78 | + * } |
| 79 | + */ |
| 80 | +/** |
| 81 | + * @param {ListNode} head |
| 82 | + * @return {number} |
| 83 | + */ |
| 84 | +var pairSum = function(head) { |
| 85 | + var num = 0; |
| 86 | + var node = head; |
| 87 | + while (node) { |
| 88 | + num++; |
| 89 | + node = node.next; |
| 90 | + } |
| 91 | + |
| 92 | + var stack = []; |
| 93 | + var sum = 0; |
| 94 | + var i = 0; |
| 95 | + while (i < num) { |
| 96 | + if (i < num / 2) { |
| 97 | + stack.push(head.val); |
| 98 | + } else { |
| 99 | + sum = Math.max(sum, head.val + stack.pop()); |
| 100 | + } |
| 101 | + i += 1; |
| 102 | + head = head.next; |
| 103 | + } |
| 104 | + |
| 105 | + return sum; |
| 106 | +}; |
| 107 | +``` |
| 108 | + |
| 109 | +**Explain:** |
| 110 | + |
| 111 | +nope. |
| 112 | + |
| 113 | +**Complexity:** |
| 114 | + |
| 115 | +* Time complexity : O(n). |
| 116 | +* Space complexity : O(n). |
0 commit comments