|
| 1 | +# 1560. Most Visited Sector in a Circular Track |
| 2 | + |
| 3 | +- Difficulty: Easy. |
| 4 | +- Related Topics: Array, Simulation. |
| 5 | +- Similar Questions: . |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]` |
| 10 | + |
| 11 | +Return **an array of the most visited sectors** sorted in **ascending** order. |
| 12 | + |
| 13 | +Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example). |
| 14 | + |
| 15 | + |
| 16 | +Example 1: |
| 17 | + |
| 18 | +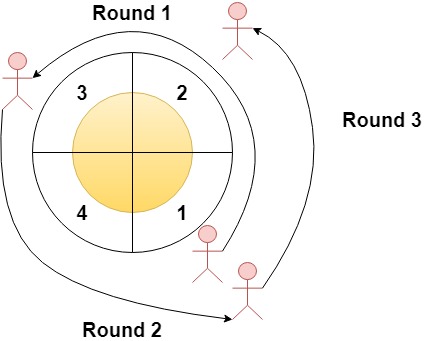 |
| 19 | + |
| 20 | +``` |
| 21 | +Input: n = 4, rounds = [1,3,1,2] |
| 22 | +Output: [1,2] |
| 23 | +Explanation: The marathon starts at sector 1. The order of the visited sectors is as follows: |
| 24 | +1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon) |
| 25 | +We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once. |
| 26 | +``` |
| 27 | + |
| 28 | +Example 2: |
| 29 | + |
| 30 | +``` |
| 31 | +Input: n = 2, rounds = [2,1,2,1,2,1,2,1,2] |
| 32 | +Output: [2] |
| 33 | +``` |
| 34 | + |
| 35 | +Example 3: |
| 36 | + |
| 37 | +``` |
| 38 | +Input: n = 7, rounds = [1,3,5,7] |
| 39 | +Output: [1,2,3,4,5,6,7] |
| 40 | +``` |
| 41 | + |
| 42 | + |
| 43 | +**Constraints:** |
| 44 | + |
| 45 | + |
| 46 | + |
| 47 | +- `2 <= n <= 100` |
| 48 | + |
| 49 | +- `1 <= m <= 100` |
| 50 | + |
| 51 | +- `rounds.length == m + 1` |
| 52 | + |
| 53 | +- `1 <= rounds[i] <= n` |
| 54 | + |
| 55 | +- `rounds[i] != rounds[i + 1]` for `0 <= i < m` |
| 56 | + |
| 57 | + |
| 58 | + |
| 59 | +## Solution |
| 60 | + |
| 61 | +```javascript |
| 62 | +/** |
| 63 | + * @param {number} n |
| 64 | + * @param {number[]} rounds |
| 65 | + * @return {number[]} |
| 66 | + */ |
| 67 | +var mostVisited = function(n, rounds) { |
| 68 | + var start = rounds[0]; |
| 69 | + var end = rounds[rounds.length - 1]; |
| 70 | + if (end >= start) { |
| 71 | + return Array(end - start + 1).fill(0).reduce((arr, num, i) => { |
| 72 | + arr.push(start + i); |
| 73 | + return arr; |
| 74 | + }, []); |
| 75 | + } else { |
| 76 | + var arr1 = Array(n - start + 1).fill(0).reduce((arr, num, i) => { |
| 77 | + arr.push(start + i); |
| 78 | + return arr; |
| 79 | + }, []); |
| 80 | + var arr2 = Array(end).fill(0).reduce((arr, num, i) => { |
| 81 | + arr.push(i + 1); |
| 82 | + return arr; |
| 83 | + }, []); |
| 84 | + return arr2.concat(arr1); |
| 85 | + } |
| 86 | +}; |
| 87 | +``` |
| 88 | + |
| 89 | +**Explain:** |
| 90 | + |
| 91 | +if start <= end, return range[start, end] |
| 92 | + |
| 93 | +else return range[0, end] + range[start, n] |
| 94 | + |
| 95 | +**Complexity:** |
| 96 | + |
| 97 | +* Time complexity : O(n). |
| 98 | +* Space complexity : O(n). |
0 commit comments