|
| 1 | +# GraphQL API with Next.js & MongoDB |
1 | 2 |
|
| 3 | +## 1. GraphQL API with Next.js & MongoDB: Access & Refresh Tokens |
| 4 | + |
| 5 | +This article will teach you how to build a GraphQL API with Next.js to implement JWT Authentication using apollo-server-micro, TypeGraphQL, MongoDB, Redis, Mongoose, and Typegoose. |
| 6 | + |
| 7 | +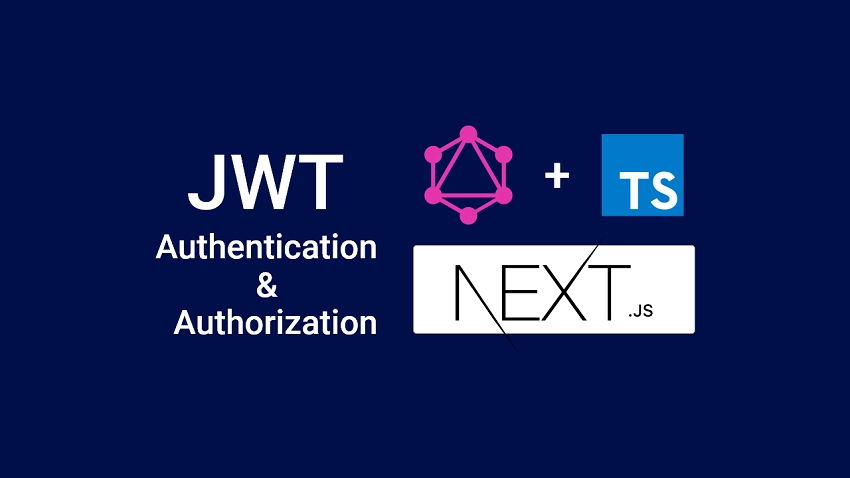 |
| 8 | + |
| 9 | +### Topics Covered |
| 10 | + |
| 11 | +- Initialize a Typescript Next.js Project |
| 12 | +- Setup MongoDB and Redis Database Servers |
| 13 | +- Setting up Environment Variables |
| 14 | +- Connect the Redis and MongoDB Servers to Next.js |
| 15 | + - Connect to the MongoDB Server |
| 16 | + - Connect to the Redis Server |
| 17 | +- Start the GraphQL Apollo Server |
| 18 | +- Creating TypeGraphQL and Typegoose Schemas |
| 19 | + - Creating the Typegoose Schema |
| 20 | + - Creating the TypeGraphQL Schemas |
| 21 | +- Creating an Error Handler |
| 22 | +- Create Utility Functions to Generate and Verify JWTs |
| 23 | + - How to Generate the Private and Public Keys |
| 24 | +- Create an Authentication Guard |
| 25 | +- Creating the Authentication Services |
| 26 | + - Service to Register the User |
| 27 | + - Service to Sign in the User |
| 28 | + - Service to Get the Authenticated User |
| 29 | + - Service to Refresh the Access Token |
| 30 | + - Service to Logout the User |
| 31 | +- Create the TypeGraphQL Resolvers |
| 32 | +- Update the GraphQL Server |
| 33 | +- Testing the GraphQL API in Postman |
| 34 | + |
| 35 | +Read the entire article here: [https://codevoweb.com/graphql-api-next-mongodb-access-and-refresh-tokens](https://codevoweb.com/graphql-api-next-mongodb-access-and-refresh-tokens) |
| 36 | + |
| 37 | +## 2. Next.js, GraphQL-CodeGen, & React Query: JWT Authentication |
| 38 | + |
| 39 | +This article will teach you how to add access and refresh token functionalities to your Next.js app using React Query, graphql-request, GraphQL CodeGen, React-Hook-Form, and Zod. |
| 40 | + |
| 41 | +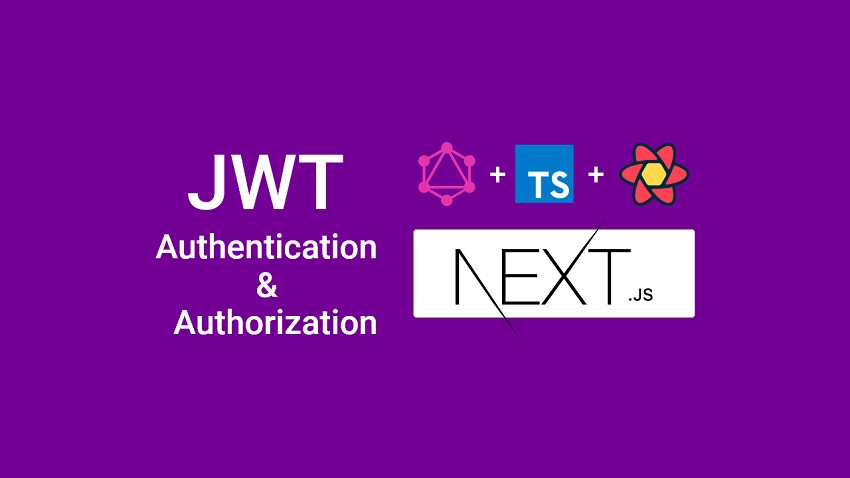 |
| 42 | + |
| 43 | +### Topics Covered |
| 44 | + |
| 45 | +- Next.js, React Query & GraphQL-CodeGen Overview |
| 46 | +- Setup React Query & GraphQL CodeGen in Next.js |
| 47 | + - Install and Setup React Query |
| 48 | + - Install GraphQL and GraphQL-Request |
| 49 | + - GraphQL-CodeGen Manual Setup |
| 50 | +- Create the GraphQL Mutations and Queries |
| 51 | + - Sign-up User Mutation |
| 52 | + - Sign-in User Mutation |
| 53 | + - Get Authenticated User Query |
| 54 | + - Refresh Access Token Query |
| 55 | + - Logout User Query |
| 56 | +- Generating the Typescript Types & React Query Hooks |
| 57 | +- Setup tailwindCss in Next.js |
| 58 | +- Creating React Query, Axios & GraphQL Clients |
| 59 | + - GraphQL Request Client |
| 60 | + - Axios GraphQL Request Client |
| 61 | +- State Management with Zustand |
| 62 | +- Creating React Components with TailwindCSS |
| 63 | + - Creating a Loading Spinner |
| 64 | + - Creating the Header Component |
| 65 | + - Creating a Full-Screen Loader |
| 66 | + - Creating a Loading Button |
| 67 | + - Creating an InputField Component with React-Hook-Form |
| 68 | + - Creating a FileUpload with Cloudinary and React |
| 69 | +- React Query & GraphQL Request: Sign-up User |
| 70 | +- React Query & GraphQL Request: Login User |
| 71 | +- React Query & GraphQL Request: Middleware Guard |
| 72 | +- Creaeting the Profile and Home Pages |
| 73 | + - Home Page |
| 74 | + - Profile Page |
| 75 | +- Update the App File |
| 76 | + |
| 77 | +Read the entire article here: [https://codevoweb.com/nextjs-graphql-codegen-react-query-jwt-authentication](https://codevoweb.com/nextjs-graphql-codegen-react-query-jwt-authentication) |
| 78 | + |
| 79 | +## 3. GraphQL CRUD API with Next.js, MongoDB, and TypeGraphQL |
| 80 | + |
| 81 | +This article will teach you how to build a GraphQL API with Next.js to implement the basic CRUD operations using apollo-server-micro, TypeGraphQL, MongoDB, Redis, Mongoose, and Typegoose. |
| 82 | + |
| 83 | +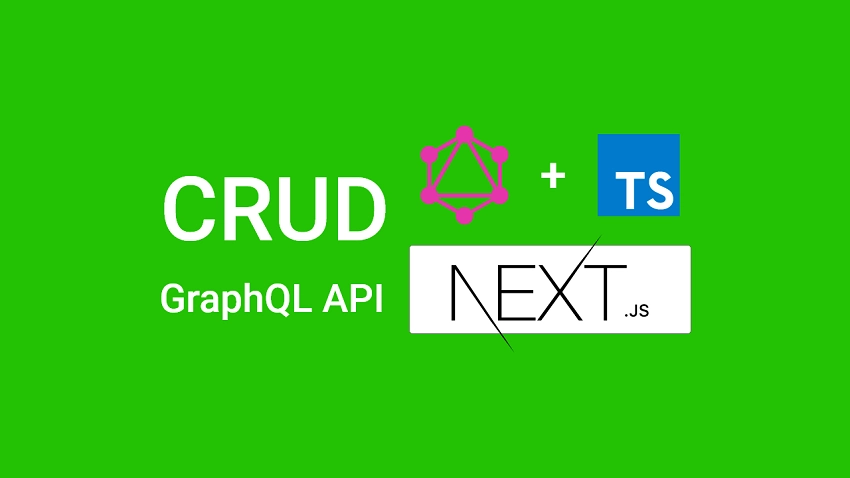 |
| 84 | + |
| 85 | +### Topics Covered |
| 86 | + |
| 87 | +- What is TypeGraphQL? |
| 88 | +- Initialize a Typescript Next.js Project |
| 89 | +- Setup MongoDB and Redis Databases |
| 90 | +- Setting up Environment Variables |
| 91 | +- Connecting to the Redis and MongoDB Databases |
| 92 | + - Connect to the MongoDB Database |
| 93 | + - Connect to the Redis Database |
| 94 | +- Setup the GraphQL Apollo Server in Next.js |
| 95 | +- Creating TypeGraphQL and Typegoose Schemas |
| 96 | + - Creating the Typegoose Schema |
| 97 | + - Creating the TypeGraphQL Schemas |
| 98 | +- Create a Global Error Handler |
| 99 | +- Creating the CRUD Services |
| 100 | + - GraphQL Create Post Service |
| 101 | + - GraphQL Get a Single Post Service |
| 102 | + - GraphQL Update Post Service |
| 103 | + - GraphQL Get all Posts Service |
| 104 | + - GraphQL Delete Post Service |
| 105 | +- Creating the CRUD TypeGraphQL Resolvers |
| 106 | +- Update the Apollo GraphQL Server |
| 107 | +- Testing the GraphQL CRUD API in Postman |
| 108 | + |
| 109 | +Read the entire article here: [https://codevoweb.com/graphql-crud-api-nextjs-mongodb-typegraphql](https://codevoweb.com/graphql-crud-api-nextjs-mongodb-typegraphql) |
| 110 | + |
| 111 | +## 4. Next.js Full-Stack App with React Query, and GraphQL-CodeGen |
| 112 | + |
| 113 | +This article will teach you how to build a full-stack CRUD App with Next.js, React Query, GraphQL Code Generator, React-Hook-Form, Zod, and graphql-request to perform Create/Update/Get/Delete operations. |
| 114 | + |
| 115 | +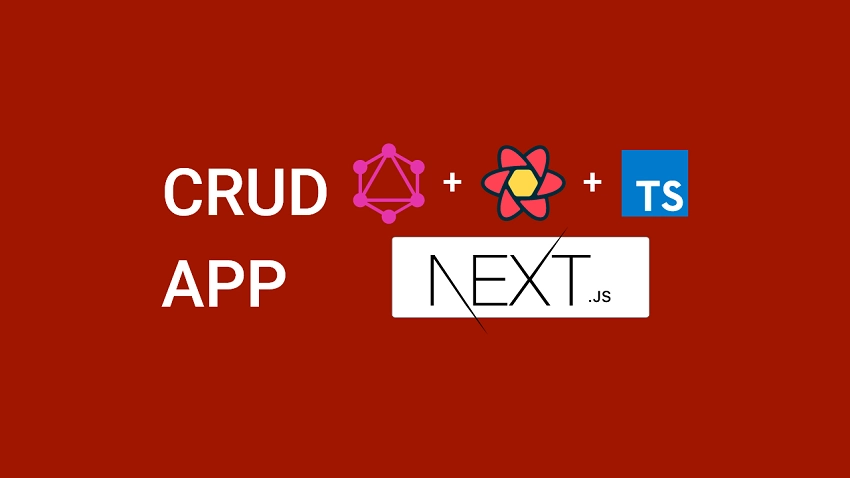 |
| 116 | + |
| 117 | +### Topics Covered |
| 118 | + |
| 119 | +- Next.js Full-Stack CRUD App Overview |
| 120 | +- Benefits of React Query |
| 121 | +- Setup GraphQL Code Generator |
| 122 | +- Creating the GraphQL Mutations and Queries |
| 123 | + - Create Post Mutation |
| 124 | + - Update Post Mutation |
| 125 | + - Delete Post Mutation |
| 126 | + - Get a Single Post Query |
| 127 | + - Get All Post Query |
| 128 | +- Generating the React Query Hooks with CodeGen |
| 129 | +- Create Reusable Components with tailwindCss |
| 130 | + - Creating the Modal Component |
| 131 | + - Creating the Message Component |
| 132 | + - Creating a Custom Input Field with React-Hook-Form |
| 133 | +- GraphQL Request and React Query Clients |
| 134 | +- React Query & GraphQL Request Create Mutation |
| 135 | +- React Query & GraphQL Request Update Mutation |
| 136 | +- React Query & GraphQL Request Delete Mutation |
| 137 | +- React Query & GraphQL Request Get Query |
| 138 | + |
| 139 | +Read the entire article here: [https://codevoweb.com/nextjs-full-stack-app-with-react-query-and-graphql-codegen](https://codevoweb.com/nextjs-full-stack-app-with-react-query-and-graphql-codegen) |
0 commit comments