|
| 1 | +## 3.5.9 |
| 2 | + |
| 3 | +❤️ Thanks all to those who contributed to make this release! ❤️ |
| 4 | + |
| 5 | +🛩️ *Features* |
| 6 | +* feat: expose WebElement (#4043) - by @KobeNguyenT |
| 7 | +``` |
| 8 | +Now we expose the WebElements that are returned by the WebHelper and you could make the subsequence actions on them. |
| 9 | +
|
| 10 | +// Playwright helper would return the Locator |
| 11 | +
|
| 12 | +I.amOnPage('/form/focus_blur_elements'); |
| 13 | +const webElements = await I.grabWebElements('#button'); |
| 14 | +webElements[0].click(); |
| 15 | +``` |
| 16 | +* feat(playwright): support HAR replaying (#3990) - by @KobeNguyenT |
| 17 | +``` |
| 18 | +Replaying from HAR |
| 19 | +
|
| 20 | + // Replay API requests from HAR. |
| 21 | + // Either use a matching response from the HAR, |
| 22 | + // or abort the request if nothing matches. |
| 23 | + I.replayFromHar('./output/har/something.har', { url: "*/**/api/v1/fruits" }); |
| 24 | + I.amOnPage('https://demo.playwright.dev/api-mocking'); |
| 25 | + I.see('CodeceptJS'); |
| 26 | +[Parameters] |
| 27 | +harFilePath [string](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String) Path to recorded HAR file |
| 28 | +opts [object](https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object)? [Options for replaying from HAR](https://playwright.dev/docs/api/class-page#page-route-from-har) |
| 29 | +``` |
| 30 | +* feat(playwright): support HAR recording (#3986) - by @KobeNguyenT |
| 31 | +``` |
| 32 | +A HAR file is an HTTP Archive file that contains a record of all the network requests that are made when a page is loaded. |
| 33 | +It contains information about the request and response headers, cookies, content, timings, and more. |
| 34 | +You can use HAR files to mock network requests in your tests. HAR will be saved to output/har. |
| 35 | +More info could be found here https://playwright.dev/docs/api/class-browser#browser-new-context-option-record-har. |
| 36 | +
|
| 37 | +... |
| 38 | +recordHar: { |
| 39 | + mode: 'minimal', // possible values: 'minimal'|'full'. |
| 40 | + content: 'embed' // possible values: "omit"|"embed"|"attach". |
| 41 | +} |
| 42 | +... |
| 43 | +``` |
| 44 | +* improvement(playwright): support partial string for option (#4016) - by @KobeNguyenT |
| 45 | +``` |
| 46 | +await I.amOnPage('/form/select'); |
| 47 | +await I.selectOption('Select your age', '21-'); |
| 48 | +``` |
| 49 | + |
| 50 | +🐛 *Bug Fixes* |
| 51 | +* fix(playwright): proceedSee could not find the element (#4006) - by @hatufacci |
| 52 | +* fix(appium): remove the vendor prefix of 'bstack:options' (#4053) - by @mojtabaalavi |
| 53 | +* fix(workers): event improvements (#3953) - by @KobeNguyenT |
| 54 | +``` |
| 55 | +Emit the new event: event.workers.result. |
| 56 | +
|
| 57 | +CodeceptJS also exposes the env var `process.env.RUNS_WITH_WORKERS` when running tests with run-workers command so that you could handle the events better in your plugins/helpers. |
| 58 | +
|
| 59 | +const { event } = require('codeceptjs'); |
| 60 | +
|
| 61 | +module.exports = function() { |
| 62 | + // this event would trigger the `_publishResultsToTestrail` when running `run-workers` command |
| 63 | + event.dispatcher.on(event.workers.result, async () => { |
| 64 | + await _publishResultsToTestrail(); |
| 65 | + }); |
| 66 | + |
| 67 | + // this event would not trigger the `_publishResultsToTestrail` multiple times when running `run-workers` command |
| 68 | + event.dispatcher.on(event.all.result, async () => { |
| 69 | + // when running `run` command, this env var is undefined |
| 70 | + if (!process.env.RUNS_WITH_WORKERS) await _publishResultsToTestrail(); |
| 71 | + }); |
| 72 | +} |
| 73 | +``` |
| 74 | +* fix: ai html updates (#3962) - by @davert |
| 75 | +``` |
| 76 | +replaced minify library with a modern and more secure fork. Fixes [email protected] Regular Expression Denial of Service vulnerability #3829 |
| 77 | +AI class is implemented as singleton |
| 78 | +refactored heal.js plugin to work on edge cases |
| 79 | +add configuration params on number of fixes performed by ay heal |
| 80 | +improved recorder class to add more verbose log |
| 81 | +improved recorder class to ignore some of errors |
| 82 | +``` |
| 83 | +* fix(appium): closeApp supports both Android/iOS (#4046) - by @KobeNguyenT |
| 84 | +* fix: some security vulnerability of some packages (#4045) - by @KobeNguyenT |
| 85 | +* fix: seeAttributesOnElements check condition (#4029) - by @KobeNguyenT |
| 86 | +* fix: waitForText locator issue (#4039) - by @KobeNguyenT |
| 87 | +``` |
| 88 | +Fixed this error: |
| 89 | +
|
| 90 | +locator.isVisible: Unexpected token "s" while parsing selector ":has-text('Were you able to resolve the resident's issue?') >> nth=0" |
| 91 | + at Playwright.waitForText (node_modules\codeceptjs\lib\helper\Playwright.js:2584:79) |
| 92 | +``` |
| 93 | +* fix: move to sha256 (#4038) - by @KobeNguyenT |
| 94 | +* fix: respect retries from retryfailedstep plugin in helpers (#4028) - by @KobeNguyenT |
| 95 | +``` |
| 96 | +Currently inside the _before() of helpers for example Playwright, the retries is set there, however, when retryFailedStep plugin is enabled, the retries of recorder is still using the value from _before() not the value from retryFailedStep plugin. |
| 97 | +
|
| 98 | +Fix: |
| 99 | +
|
| 100 | +- introduce the process.env.FAILED_STEP_RETIRES which could be access everywhere as the helper won't know anything about the plugin. |
| 101 | +- set default retries of Playwright to 3 to be on the same page with Puppeteer. |
| 102 | +``` |
| 103 | +* fix: examples in test title (#4030) - by @KobeNguyenT |
| 104 | +``` |
| 105 | +When test title doesn't have the data in examples: |
| 106 | +
|
| 107 | +Feature: Faker examples |
| 108 | +
|
| 109 | + Scenario Outline: Below are the users |
| 110 | + Examples: |
| 111 | + | user | role | |
| 112 | + | John | admin | |
| 113 | + | Tim | client | |
| 114 | +
|
| 115 | +Faker examples -- |
| 116 | + [1] Starting recording promises |
| 117 | + Timeouts: |
| 118 | + Below are the users {"user":"John","role":"admin"} |
| 119 | + ✔ OK in 4ms |
| 120 | +
|
| 121 | + Below are the users {"user":"Tim","role":"client"} |
| 122 | + ✔ OK in 1ms |
| 123 | +
|
| 124 | +When test title includes the data in examples: |
| 125 | +
|
| 126 | +
|
| 127 | +Feature: Faker examples |
| 128 | +
|
| 129 | + Scenario Outline: Below are the users - <user> - <role> |
| 130 | + Examples: |
| 131 | + | user | role | |
| 132 | + | John | admin | |
| 133 | + | Tim | client | |
| 134 | +
|
| 135 | +
|
| 136 | +Faker examples -- |
| 137 | + [1] Starting recording promises |
| 138 | + Timeouts: |
| 139 | + Below are the users - John - admin |
| 140 | + ✔ OK in 4ms |
| 141 | +
|
| 142 | + Below are the users - Tim - client |
| 143 | + ✔ OK in 1ms |
| 144 | +``` |
| 145 | +* fix: disable retryFailedStep when using with tryTo (#4022) - by @KobeNguyenT |
| 146 | +* fix: locator builder returns error when class name contains hyphen (#4024) - by @KobeNguyenT |
| 147 | +* fix: seeCssPropertiesOnElements failed when font-weight is a number (#4026) - by @KobeNguyenT |
| 148 | +* fix(appium): missing await on some steps of runOnIOS and runOnAndroid (#4018) - by @KobeNguyenT |
| 149 | +* fix(cli): no error of failed tests when using retry with scenario only (#4020) - by @KobeNguyenT |
| 150 | +* fix: set getPageTimeout to 30s (#4031) - by @KobeNguyenT |
| 151 | +* fix(appium): expose switchToContext (#4015) - by @KobeNguyenT |
| 152 | +* fix: promise issue (#4013) - by @KobeNguyenT |
| 153 | +* fix: seeCssPropertiesOnElements issue with improper condition (#4057) - by @KobeNguyenT |
| 154 | + |
| 155 | +📖 *Documentation* |
| 156 | +* docs: Update clearCookie documentation for Playwright helper (#4005) - by @Hellosager |
| 157 | +* docs: improve the example code for autoLogin (#4019) - by @KobeNguyenT |
| 158 | + 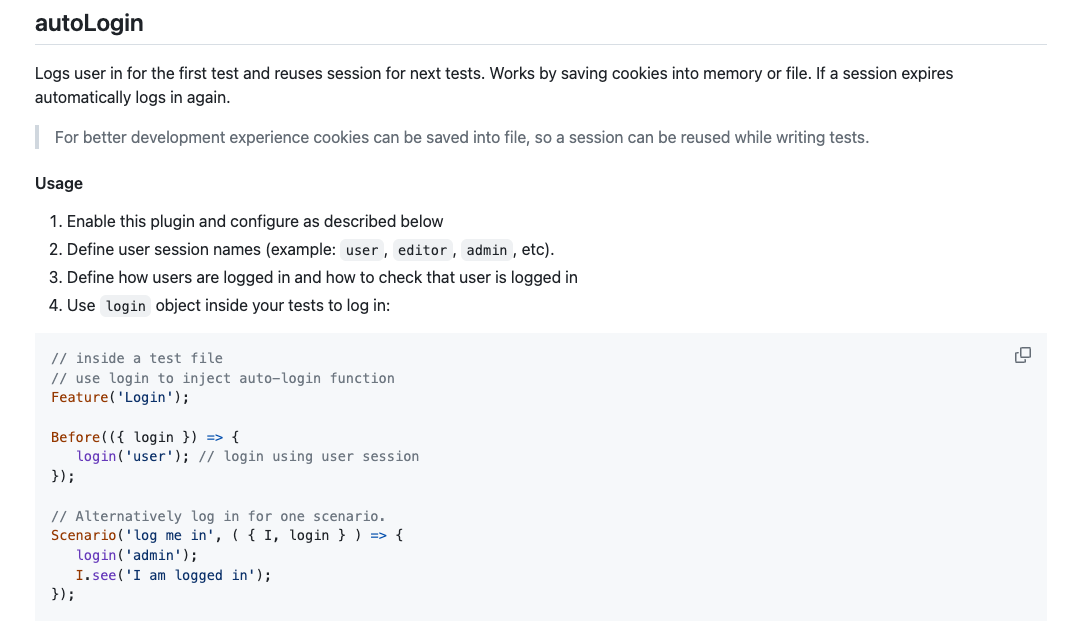 |
| 159 | + |
1 | 160 | ## 3.5.8
|
2 | 161 |
|
3 | 162 | Thanks all to those who contributed to make this release!
|
4 | 163 |
|
5 | 164 | 🐛 *Bug Fixes*
|
6 | 165 | fix(appium): type of setNetworkConnection() (#3994) - by @mirao
|
7 | 166 | fix: improve the way to show deprecated appium v1 message (#3992) - by @KobeNguyenT
|
8 |
| -fix: missing exit condition of some wait functions - by @kobenguyent |
| 167 | +fix: missing exit condition of some wait functions - by @KobeNguyenT |
9 | 168 |
|
10 | 169 | ## 3.5.7
|
11 | 170 |
|
|
0 commit comments