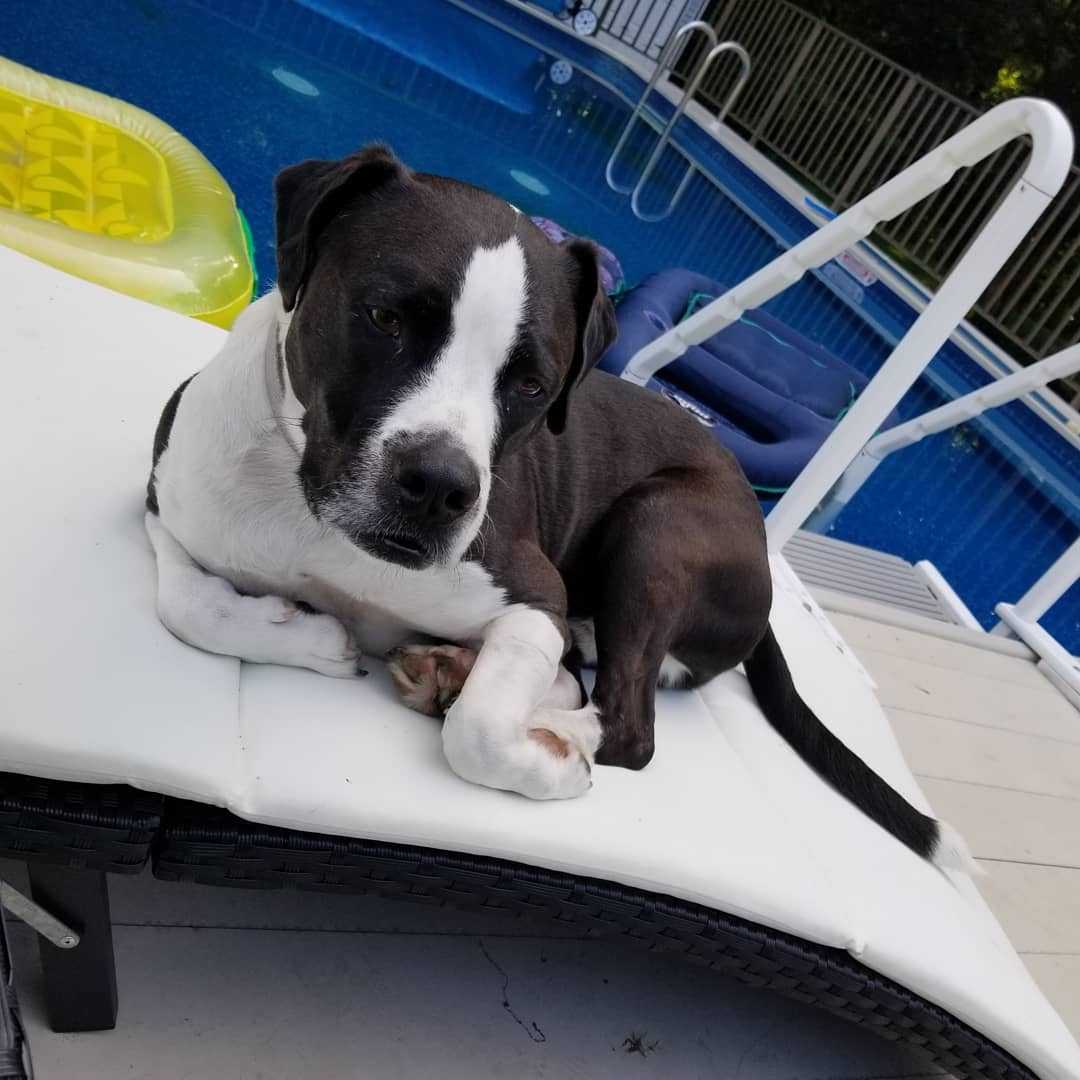
+```javascript
// Declare a Node() function that we will use to instantiate new Node objects.
function Node(data) {
- this.data = data;
- this.next = null;
+ this.data = data;
+ this.next = null;
}
// Declare a SinglyLinkedList() function that we will use as a basis for our singly-linked list.
function SinglyLinkedList() {
-this.\_length = 0;
-this.head = null;
+ this._length = 0;
+ this.head = null;
}
// Use JavaScript prototyping to give the SinglyLinkedList class new public methods.
-SinglyLinkedList.prototype.add = function(value) {
-let node = new Node(value),
-currentNode = this.head;
+SinglyLinkedList.prototype.add = function (value) {
+ let node = new Node(value),
+ currentNode = this.head;
- // If the list is empty (has no head value)
- if (!currentNode) {
- this.head = node;
- this._length++;
-
- return node;
- }
-
- // Loop over all nodes that have a value in their "next" property.
- // This loop ends when it reaches a node that has no value in the "next" property.
- // We use this to determine the "last" node of the singly linked list.
- while (currentNode.next) {
- currentNode = currentNode.next;
- }
-
- // We can now add our node to the end of the list by storing it in the "next" of the node we determined was last in the list.
- currentNode.next = node;
-
- // We need to increment the length of the list now that we've added a new node.
+ // If the list is empty (has no head value)
+ if (!currentNode) {
+ this.head = node;
this._length++;
return node;
+ }
-};
-
-SinglyLinkedList.prototype.findByPosition = function(position) {
-let currentNode = this.head,
-length = this.\_length,
-count = 1,
-message = {failure: 'Failure: non-existent node in this list.'};
+ // Loop over all nodes that have a value in their "next" property.
+ // This loop ends when it reaches a node that has no value in the "next" property.
+ // We use this to determine the "last" node of the singly linked list.
+ while (currentNode.next) {
+ currentNode = currentNode.next;
+ }
- // Catch the possibility that a position that doesn't exist was provided.
- if (length === 0 || position < 1 || position > length) {
- throw new Error(message.failure);
- }
+ // We can now add our node to the end of the list by storing it in the "next" of the node we determined was last in the list.
+ currentNode.next = node;
- // Loop over all nodes until the node before the desired position
- while (count < position) {
- // Pull the "next" node object from the node based on the count
- currentNode = currentNode.next;
- count++;
- }
+ // We need to increment the length of the list now that we've added a new node.
+ this._length++;
- // Because our loop stopped at the position before, our "currentNode" value is correctly set.
- return currentNode;
+ return node;
+};
+SinglyLinkedList.prototype.findByPosition = function (position) {
+ let currentNode = this.head,
+ length = this._length,
+ count = 1,
+ message = { failure: 'Failure: non-existent node in this list.' };
+
+ // Catch the possibility that a position that doesn't exist was provided.
+ if (length === 0 || position < 1 || position > length) {
+ throw new Error(message.failure);
+ }
+
+ // Loop over all nodes until the node before the desired position
+ while (count < position) {
+ // Pull the "next" node object from the node based on the count
+ currentNode = currentNode.next;
+ count++;
+ }
+
+ // Because our loop stopped at the position before, our "currentNode" value is correctly set.
+ return currentNode;
};
-SinglyLinkedList.prototype.remove = function(position) {
-let currentNode = this.head,
-length = this.\_length,
-count = 0,
-message = {failure: 'Failure: non-existent node in this list.'},
-beforeNodeToDelete = null,
-nodeToDelete = null,
-deletedNode = null;
-
- // Catch the possibility that a position that doesn't exist was provided.
- if (position < 0 || position > length) {
- throw new Error(message.failure);
- }
-
- // Only run when the first node is being removed.
- if (position === 1) {
- this.head = currentNode.next;
- deletedNode = currentNode;
- currentNode = null;
- this._length--;
-
- return deletedNode;
- }
-
- // Remaining logic that is run when any node is being removed.
- while (count < position) {
- beforeNodeToDelete = currentNode;
- nodeToDelete = currentNode.next;
- count++;
- }
-
- beforeNodeToDelete.next = nodeToDelete.next;
- deletedNode = nodeToDelete;
- nodeToDelete = null;
+SinglyLinkedList.prototype.remove = function (position) {
+ let currentNode = this.head,
+ length = this._length,
+ count = 0,
+ message = { failure: 'Failure: non-existent node in this list.' },
+ beforeNodeToDelete = null,
+ nodeToDelete = null,
+ deletedNode = null;
+
+ // Catch the possibility that a position that doesn't exist was provided.
+ if (position < 0 || position > length) {
+ throw new Error(message.failure);
+ }
+
+ // Only run when the first node is being removed.
+ if (position === 1) {
+ this.head = currentNode.next;
+ deletedNode = currentNode;
+ currentNode = null;
this._length--;
return deletedNode;
+ }
+
+ // Remaining logic that is run when any node is being removed.
+ while (count < position) {
+ beforeNodeToDelete = currentNode;
+ nodeToDelete = currentNode.next;
+ count++;
+ }
+ beforeNodeToDelete.next = nodeToDelete.next;
+ deletedNode = nodeToDelete;
+ nodeToDelete = null;
+ this._length--;
+
+ return deletedNode;
};
-
+```
---
@@ -184,9 +181,10 @@ Create a method to add a new node after the node with the text attribute matchin
See [Testing and TDD](../testing-and-tdd/testing-and-tdd.md) for a refresher on how to use Mocha and Chai to write tests.
Create a file called "LinkedList_test.js" and write tests for each of your methods using Mocha and Chai be sure to include:
-
+
+```js
const LinkedList = require('./linkedlist.js');
-
+```
### Check for Understanding
diff --git a/data-structures/queue-screenshots/queue.png b/data-structures/queue-screenshots/queue.png
new file mode 100644
index 000000000..50d8d3ad1
Binary files /dev/null and b/data-structures/queue-screenshots/queue.png differ
diff --git a/data-structures/queues.md b/data-structures/queues.md
index 28886dbbd..4e3a6ce11 100644
--- a/data-structures/queues.md
+++ b/data-structures/queues.md
@@ -13,18 +13,13 @@ About 60-90 minutes
### Prerequisites
- [What is a Data Structure](/data-structures/intro-to-data-structures.md)
-- [JavaScript 1 - Variables, Strings, Numbers](/javascript/javascript-1-variables.md)
-- [JavaScript 2 - Arrays, Functions](/javascript/javascript-2-arrays-functions.md)
-- [JavaScript 3 - Conditionals, Comparisons, Booleans](/javascript/javascript-3-conditionals.md)
-- [JavaScript 4 - Loops](/javascript/javascript-4-loops.md)
-- [JavaScript 5 - Switch Statements](/javascript/javascript-5-switch.md)
-- [JavaScript 6 - Object Literals](/javascript/javascript-6-object-literals.md)
-- [JavaScript 7 - Object-Oriented Programming](/javascript/javascript-7-oop.md)
- [Runtime Complexity](/runtime-complexity/runtime-complexity.md)
### Motivation
-Queues are widely used both in programming and in real life. In programming: if you have an array of tasks to execute and not enough time to do them all as they come in, you'll need some way to both track the tasks and execute them in the order they arrived. In real life: in line for a coffee, or on hold for customer support -- there are many everyday scenarios that are real-life implementations of queues.
+Queues are widely used both in programming and in real life. _In programming_: if you have an array of tasks to execute and not enough time to do them all as they come in, you'll need some way to both track the tasks and execute them in the order they arrived. _In real life_: queue can be a single-lane one-way road, where the vehicle enters first, exits first. More real-world examples can be seen as queues at the ticket windows and bus-stops- there are many everyday scenarios that are real-life implementations of queues.
+
+
Application of queue in computer science:
@@ -50,22 +45,155 @@ Application of queue in computer science:
### Materials
-- [Queue Slides](https://docs.google.com/presentation/d/1nBWaTq5Sm1EKbquW12LmonMkW6OqAUGpQI1nW6fiNWI/edit?usp=sharing)
+- [Queue Slides](https://docs.google.com/presentation/d/1-jcSrAvf6Bnaa-vV9uhGx2x3Zxoi5-EaEN7ybx3M4Kc/edit#slide=id.g3c8d44f31f_0_54)
- [A Gentle Introduction to Data Structures: How Queues Work (20 mins read)](https://medium.freecodecamp.org/a-gentle-introduction-to-data-structures-how-queues-work-f8b871938e64)
- [Introduction to queues video (10 mins watch)](https://www.youtube.com/watch?v=XuCbpw6Bj1U)
### Lesson
-1. Read through lesson slides [Queue](https://docs.google.com/presentation/d/1nBWaTq5Sm1EKbquW12LmonMkW6OqAUGpQI1nW6fiNWI/edit?usp=sharing).
+1. Read through lesson slides [Queue](https://docs.google.com/presentation/d/1-jcSrAvf6Bnaa-vV9uhGx2x3Zxoi5-EaEN7ybx3M4Kc/edit#slide=id.g3c8d44f31f_0_54).
2. Watch the video and read the article in the materials section.
+#### What is Queue?
+
+A queue is an ordered list of elements where an element is inserted at the end of the queue and is removed from the front of the queue.
+
+A queue follows the _First-In First-Out (FIFO)_ paradigm: the first item added will be the first item removed.
+
+#### Implementation of Queue in Javascript
+
+A queue can be implemented in javascript with the help of an array object.
+
+**Basic Operations**
+
+- `enqueue()` - add new element(s) to the queue;
+- `dequeue()` - remove first element from the queue;
+- `front() or peek()` - returns the first element from the queue without removing it.(for checking purposes);
+- `isEmpty()` - returns if the queue is empty or not;
+- `getLength()` - returns how many elements the queue contains.
+
+### Guided Practice
+
+Let’s create a MyQueue constructor
+
+```js
+// create a constructor function called myQueue
+function MyQueue() {
+ //array to store its elements.
+ this.elements = [];
+}
+```
+
+The `enqueue()` method add elements to a queue at the last position or index. The [push()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/push) method can be used to implement enqueue.
+
+```js
+MyQueue.prototype.enqueue = function (value) {
+ return this.elements.push(value);
+};
+```
+
+The `dequeue()` method remove the first element from the queue and return it. The [shift()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/shift) method can be used to implement dequeue.
+
+```js
+MyQueue.prototype.dequeue = function () {
+ return this.elements.shift();
+};
+```
+
+> **_NOTE:_** Time complexity of _Enqueue_ and _Dequeue_ is O(1).
+
+The `peek()` method looks at the first element without popping it from the queue.
+
+```js
+MyQueue.prototype.peek = function () {
+ return this.elements[0];
+};
+```
+
+The `isEmpty()` method checks if a queue is empty and returns true if the queue is empty. we can implement by checking if the length property of the array is zero.
+
+```js
+MyQueue.prototype.isEmpty = function () {
+ return this.elements.length === 0;
+};
+```
+
+The `getLength()` method returns the length of a queue object.
+
+```js
+MyQueue.prototype.getLength = function () {
+ return this.elements.length;
+};
+```
+
+You can use other method to implement Queue in JS. Here is another example using `Class` constructor.
+
+```js
+class MyQueue {
+ constructor() {
+ this.elements = [];
+ }
+ // add new element
+ enqueue(e) {
+ return this.elements.push(e);
+ }
+ // remove first element
+ dequeue() {
+ return this.elements.shift();
+ }
+ // get the length of queue
+ getLength() {
+ return this.elements.length;
+ }
+ // checks if it is empty
+ isEmpty() {
+ return this.elements.length === 0;
+ }
+ // get the first element
+ peek() {
+ return this.elements[0];
+ }
+}
+```
+
+Now let’s use the MyQueue function and its different method described above
+
+```js
+// create an instance of MyQueue
+const queue1 = new MyQueue();
+
+console.log(queue1); // {elements: []}
+
+const queue1 = new MyQueue();
+
+console.log(queue1.isEmpty()); // true
+
+queue1.enqueue(1);
+queue1.enqueue(2);
+queue1.enqueue(3);
+
+console.log(queue1); // {elements: [1, 2, 3]}
+
+console.log(queue1.dequeue()); // 1
+
+console.log(queue1); // {elements: [2, 3]}
+
+console.log(queue1.peek()); // 2
+
+console.log(queue1.getLength()); // 2
+
+console.log(queue1.isEmpty()); // false
+```
+
+You can see completed code on [codepen](https://codepen.io/SupriyaRaj/pen/qBVaLyr?editors=1111)
+
### Common Mistakes / Misconceptions
- There may be an implementation that is very simple, but untenable for larger queues.
### Guided Practice
-Discuss as a group how a queue differs from other data structures already reviewed. Some suggested questions to consider:
+Discuss with your pair group for the day how a queue differs from other data structures already reviewed. Some suggested questions to consider:
- What are the methods a queue must have?
- What can be tracked in a queue?
diff --git a/data-structures/stack.md b/data-structures/stack.md
index 0e6913a7b..011df6843 100644
--- a/data-structures/stack.md
+++ b/data-structures/stack.md
@@ -38,10 +38,12 @@ Explain what a stack data structure is and show how it is implemented.
[Lesson slides](https://docs.google.com/presentation/d/1lOqqqXF-NYzFw0Cu3vIa-dLZeERGhcg1SFmOgW4Y62w/edit#slide=id.p)
[Lesson video](https://drive.google.com/open?id=1ioFhuH4I0J5gAnwyw6SJxWzioAWKNrZp)
-Make sure to mention these things:
+### What is Stack?
-- Explain what LIFO and FILO means.
-- Differentiate stack and queue.
+A stack is a basic linear data structure, in which the insertion and deletion of items happens at one end called top of the stack. It follows the order of _LIFO (last in first out)_ or _FILO (first in last out)_, the last element added to the stack will be the first element removed from the stack. The classic real-life example for stack is the stack of plates in a buffet, the plate at the top is always the first one to be removed.[^1]
+
+[](https://www.programiz.com/dsa/stack)
+_[Photo credit: programiz](https://www.programiz.com/dsa/stack)_
### Common Mistakes / Misconceptions
@@ -56,22 +58,95 @@ Explain and discuss as a class the steps involved in writing a stack structure,
- Pop/Dequeue
- Size control
-### Independent Practice
+```js
+// program to implement stack data structure
+
+// Class stack is declared to initialize an array that will be used to store items of the stack:
+class Stack {
+ constructor() {
+ this.items = [];
+ }
+
+ // add element to the stack
+ push(element) {
+ return this.items.push(element);
+ }
+
+ // removes the last item added in the stack:
+ pop() {
+ if (this.items.length > 0) {
+ return this.items.pop();
+ }
+ }
+
+ // Get the topmost element of the stack
+ peek() {
+ return this.items[this.items.length - 1];
+ }
+
+ // checks whether or not the stack is empty
+ isEmpty() {
+ return this.items.length == 0;
+ }
+
+ // the size of the stack
+ size() {
+ return this.items.length;
+ }
+
+ // empty the stack
+ clear() {
+ this.items = [];
+ }
+}
+```
+
+You can test the code by creating a new object of Stack class instance and call the methods from it:
+
+```js
+let stack = new Stack();
+stack.push(1);
+stack.push(2);
+stack.push(3);
+stack.push(4);
+console.log(stack.items); // [ 1, 2, 3, 4 ]
+
+console.log(stack.pop()); // 4
+
+console.log(stack.items); // [ 1, 2, 3]
+
+console.log(stack.peek()); // 3
-Try to write a Stack class with the steps discussed as methods:
+console.log(stack.isEmpty()); // false
+console.log(stack.size()); // 3
+
+stack.clear();
+console.log(stack.items); // []
```
-const Stack = function() {}
- // Constructor
- // Push
+Runtime Complexity of push() and pop() is Constant time, since we are using the built-in [Array.push](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/push) and [Array.pop](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/pop). Both have a runtime of O(1).
+
+### Independent Practice
+
+Try to reverse a string using a JavaScript stack
- // Pop
+```js
+function reverse(str) {
+ let stack = [];
+ // push letter into stack
- // Size management of stack
+ // implement code here....
- // Output of stack
+ // pop letter from the stack
+ let reverseStr = '';
+
+ // implement code here....
+
+ return reverseStr;
}
+
+console.log(reverse('I Love Stack')); // kcatS evoL I
```
### Challenge / Check for Understanding
@@ -93,3 +168,5 @@ Next, ask each other the following questions:
- [GeeksforGeeks: Implementation of Stack in JavaScript](https://www.geeksforgeeks.org/implementation-stack-javascript/)
- [InitJS: Implement a Stack in JavaScript](https://initjs.org/data-structure-stack-in-javascript-714f45dbf889)
+
+ [^1]: https://betterprogramming.pub/stacks-in-javascript-d2f0e4404eac
diff --git a/data-structures/stack.png b/data-structures/stack.png
new file mode 100644
index 000000000..d31cfb35f
Binary files /dev/null and b/data-structures/stack.png differ
diff --git a/data-structures/trees.md b/data-structures/trees.md
index e0d367b2b..c59210057 100644
--- a/data-structures/trees.md
+++ b/data-structures/trees.md
@@ -11,7 +11,7 @@ about 2 hours
- [Intro to Data Structures](/data-structures/intro-to-data-structures.md)
- [JavaScript 1 - Variables, Strings, Numbers](/javascript/javascript-1-variables.md)
-- [JavaScript 2 - Arrays, Functions](/javascript/javascript-2-arrays-functions.md)
+- [JavaScript 2 - Arrays, Functions](/javascript/javascript-2-array-functions.md)
- [JavaScript 3 - Conditionals, Comparisons, Booleans](/javascript/javascript-3-conditionals.md)
- [JavaScript 4 - Loops](/javascript/javascript-4-loops.md)
- [JavaScript 5 - Switch Statements](/javascript/javascript-5-switch.md)
diff --git a/databases/install-postgres-with-docker.md b/databases/install-postgres-with-docker.md
new file mode 100644
index 000000000..fd5b80862
--- /dev/null
+++ b/databases/install-postgres-with-docker.md
@@ -0,0 +1,208 @@
+# Install and Run PostgreSQL using Docker
+
+### Projected Time
+
+Total: 1 hour 30 min
+
+- Install Docker: 15 min
+- Install and Configure PSQL using Docker: 20-30 min
+- Install PG-admin using Docker: 20-30 min
+- Check for Understanding: 10 min
+
+### Prerequisites
+
+- Docker
+
+### Motivation
+
+- Installing software is hard. And it has nothing to do with your expertise as a developer. We have all seen our fair share of version clashes, esoteric build failure messages and missing dependency errors each time we embarked upon the task of installing a new software to use. We have spent countless hours copy pasting snippets of code from Stack Overflow onto our terminal and running them with the hope that one of them will magically resolve install issues and make the software run. The result is mostly despair, frustration and loss of productivity.
+- Docker provides a way out of this mess by reducing the task of installing and running software to as little as two commands (docker run and docker pull). In this lesson we will see this process in action by taking a step by step look at how easy and simple it is to setup a Postgres installation with docker.[^1]
+
+### Objectives
+
+**Participants will be able to:**
+
+- Install Docker
+- Build & run a PostgreSQL and PGAdmin using Docker
+
+### Install Docker
+
+Follow the [Docker installation](https://www.docker.com/get-started) process according to your operating system.
+
+Try running this command on terminal, if docker is installed, you should get a error free response:
+
+```sh
+$ docker --version
+```
+
+### Install and Configure PSQL using Docker
+
+#### 1. Getting the Postgres Docker Image
+
+To pull down an image from the [Postgres docker hub](https://hub.docker.com/_/postgres/?tab=description), simply run
+
+```sh
+$ docker pull postgres
+```
+
+After downloading the image you can check that is available to use:
+
+```sh
+$ docker images
+```
+
+#### 2. Create a Directory
+
+We will create a local folder and mount it as a data volume for our running container to store all the database files in a known location for you.
+
+```sh
+$ mkdir ${HOME}/postgres-data/
+```
+
+#### 3. Run the Postgres Container
+
+Starting the Postgres container is as simple as running the docker run command
+
+```sh
+$ docker run -d --name dev-postgres -e POSTGRES_PASSWORD=Password -v ${HOME}/postgres-data/:/var/lib/postgresql/data -p 5432:5432 postgres
+```
+
+- -d: Launches the container in detached mode or in other words, in the background.
+
+- -- name: An identifying name for the container. We can choose any name we want.
+
+- -e: Expose environment variable of name POSTGRES_PASSWORD with value docker to the container. This environment variable sets the superuser password for PostgreSQL. We can set POSTGRES_PASSWORD to anything we like.
+
+- -v: Mount ${HOME}/postgres-data/:/var/lib/postgresql/data on the host machine to the container side volume path /var/lib/postgresql/data created inside the container. This ensures that postgres data persists even after the container is removed.
+
+- -p: Bind port 5432 on localhost to port 5432 within the container. This option enables applications running out side of the container to be able to connect to the Postgres server running inside the container.
+
+You can verify the container is running using
+
+```sh
+$ docker ps
+```
+
+#### 4. Connect to psql
+
+Run this command to access our running container `dev-postgres`
+
+```sh
+$ docker exec -it dev-postgres bash
+```
+
+Great, now you are in the container's bash console. To connect with our “Dockerized” Postgres instance run this command
+
+```sh
+$ psql -h localhost -U postgres
+```
+
+To check the list of database
+
+```
+\l
+```
+
+To check the current date
+
+```sql
+SELECT * from CURRENT_DATE;
+```
+
+Congradulation. If you don't get any error that means we have successfully run PostgreSQL in Docker. Press `CTRL+D` to exit.
+
+### Install PG-admin using Docker(Optional)
+
+[pgAdmin](https://www.pgadmin.org/) is the most popular and feature-rich Open Source administration and development platform for PostgreSQL. You will use it to manage the DB instance as well as to run your queries against the tables of it.
+
+You will be using this [docker image](https://hub.docker.com/r/dpage/pgadmin4/) to deploy it in a container. To get the image and run the instance of the image with the following commands[^2]
+
+```sh
+docker pull dpage/pgadmin4
+```
+
+Once the image is available in your local, run the below command to create a pgAdmin container.
+
+```sh
+$ docker run -p 80:80 -e 'PGADMIN_DEFAULT_EMAIL=user@domain.local' -e 'PGADMIN_DEFAULT_PASSWORD=password' --name dev-pgadmin -d dpage/pgadmin4
+```
+
+The parameters that we are passing to the docker run command are:
+
+- -p 80:80: This parameter tells docker to map the port 80 in the container to port 80 in your computer (Docker host)
+- -e 'PGADMIN_DEFAULT_EMAIL: Environment variable for default user’s email, you will use this to log in the portal afterwards
+- -e 'PGADMIN_DEFAULT_PASSWORD': Environment variable for default user’s password
+- -d: This parameters tells docker to start the container in detached mode
+ dpage/pgadmin4: This parameter tells docker to use the image that we have previously downloaded
+
+> __PS:__ If the command fails to run due with error `port is already allocated`, Open docker dashboard and stop the container that is running on port 80.
+
+Let’s check that the container is up and running,
+
+```sh
+$ docker ps
+```
+
+To look for the IP address of the PostgreSQL container on our host, you can run this command for it:
+
+```sh
+$ docker inspect dev-postgres -f "{{json .NetworkSettings.Networks }}"
+```
+
+- docker inspect: return low-level information of Docker objects, in this case, the ‘dev-postgres’ instance’s IP Adress.
+- -f: parameter is to format the output as a JSON given a Go template.
+
+The output should look like this:
+
+```json
+{
+ "bridge": {
+ "IPAMConfig": null,
+ "Links": null,
+ "Aliases": null,
+ "NetworkID": "9d28fc17d1131317a774c6494b1b4e40eb3f889afa52b03848f48aa6d0684a28",
+ "EndpointID": "36176d4730e4eeff639ba65fc58cb853fac30ad207ab1e15c32a925fb4aca2ef",
+ "Gateway": "172.17.0.1",
+ "IPAddress": "172.17.0.3",
+ "IPPrefixLen": 16,
+ "IPv6Gateway": "",
+ "GlobalIPv6Address": "",
+ "GlobalIPv6PrefixLen": 0,
+ "MacAddress": "02:42:ac:11:00:03",
+ "DriverOpts": null
+ }
+}
+```
+
+Copy the IPAddress value into the clipboard, which is 172.17.0.3 in this example, you will need to define the connection in the pgAdmin tool.
+
+The next step is to go to your web browser and type http://localhost:80.
+
+Login pgAdmin using email address and password. In our case Email- `user@domain.local` Password - `password`
+
+Once you login click on **Add New Server**
+
+Enter the credentials to save and manage PSQL via GUI.
+**Host** - The IP address of your machine
+**Password** - Password used while creating the PSQL server with docker
+
+Once you have created the connection you should see the server on the right side of your screen. At this moment you are ready to start building your databases and tables, uploading data and querying for your analysis or applications.
+
+### Check for Understanding
+
+- Why should we use Postgres with Docker?
+
+- What is Docker image?
+
+- What is Docker container?
+
+### Supplemental Materials
+
+- [Local Development Set-Up of PostgreSQL with Docker](https://towardsdatascience.com/local-development-set-up-of-postgresql-with-docker-c022632f13ea).
+
+- [How To Install and Run PostgreSQL using Docker?](https://dev.to/shree_j/how-to-install-and-run-psql-using-docker-41j2)
+
+- [Local Development Set-Up of PostgreSQL with Docker](https://www.youtube.com/watch?v=LN9vaSZQi0U) - Video
+
+[^1]: https://hackernoon.com/dont-install-postgres-docker-pull-postgres-bee20e200198
+[^2]: https://towardsdatascience.com/local-development-set-up-of-postgresql-with-docker-c022632f13ea
diff --git a/databases/installing-postgresql.md b/databases/installing-postgresql.md
index 7e14659f4..2b9898736 100644
--- a/databases/installing-postgresql.md
+++ b/databases/installing-postgresql.md
@@ -10,15 +10,94 @@
- Use PostgreSQL from the command line (`psql`) and, optionally, a GUI admin app
-### Install PostgreSQL on Mac
-
-- Follow the [Postgres Homebrew Instructions](https://wiki.postgresql.org/wiki/Homebrew)
-- This creates a superuser named postgres
-- If you run `psql` with no args, it assumes you want to connect as your current user (`whoami` command output)
-- Run `psql postgres` to connect as the superuser
-- `create database - {this.props.color} -
-{props.color}
-- {this.props.color} -
-{props.color}
-