-
Notifications
You must be signed in to change notification settings - Fork 73
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Math domain error #12
Comments
Hi, I kept studying that error and after some 'debugging' in the raw2temp module, I discovered that my thermal_np_array had some 0 values. I'm assuming that these are some hardware bad detections and I'm replacing those 0s with some non zero value from the same row (e.g, the first non zero value). I'm including this piece of code for row in thermal_np:
if 0 in row:
non_zeros = row[row != 0]
row[row == 0] = non_zeros[0] Update 18-06-21. That method led to bad coloured images. I ended up adding the following module. def zeros_filter(matrix, row, col):
"""
Input: bidimensional np.array, row and column where the 0 lies.
Output: average of the surrounding values.
"""
shape = np.shape(matrix)
rows = shape[0]
cols = shape[1]
# Surroundings depends on the position of the element (corners, edges or inner
# points). Each 'if/elif' is for each possible position in the matrix. Surrounding
# non-zero elements are selected for the interpolation and if there isn't any, a
# non-zero value from the row is taken.
if (row not in {0,rows-1}) and (col not in {0,cols-1}): # inner points
surr = np.array([matrix[row-1][col-1],matrix[row-1][col],matrix[row][col+1],
matrix[row][col-1],matrix[row][col+1],
matrix[row+1][col-1],matrix[row+1][col],matrix[row+1][col+1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
elif (row == 0): # top row
if (col == 0):
surr = np.array([matrix[row][col+1],matrix[row+1][col],
matrix[row+1][col+1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
elif (col == cols-1):
surr = np.array([matrix[row][col-1],matrix[row+1][col],
matrix[row+1][col-1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
else:
surr = np.array([matrix[row][col-1],matrix[row][col+1],
matrix[row+1][col-1],matrix[row+1][col],matrix[row+1][col+1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
elif (row == rows-1): # bottom row
if (col == 0):
surr = np.array([matrix[row][col+1],matrix[row-1][col],
matrix[row][col+1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
elif (col == cols-1):
surr = np.array([matrix[row][col-1],matrix[row-1][col],
matrix[row-1][col-1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
else:
surr = np.array([matrix[row-1][col-1],matrix[row-1][col],matrix[row][col+1],
matrix[row][col-1],matrix[row][col+1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
elif (col == 0): # first column
surr = np.array([matrix[row-1][col],matrix[row][col+1],
matrix[row][col+1],
matrix[row+1][col],matrix[row+1][col+1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
elif (col == cols-1): # last column
surr = np.array([matrix[row-1][col],matrix[row][col-1],
matrix[row][col-1],
matrix[row+1][col],matrix[row+1][col-1]])
surr = surr[surr != 0]
if len(surr) == 0:
new_value = matrix[row][matrix[row] != 0][0]
else:
mean = np.mean(surr)
new_value = mean
return new_value I think variable names and comments are clear enough. It is some kind of "convolution". If someone knows how to do it in a easier way I'd appreciate it :) |
Hi,
I'm using this code to process some images from the FLIR i7 camera. I know that theoretically the library doesn't support that camera, but after some handwork with exiftool and images from the camera, I realized that I could use it for extract the thermal_np_array which is enough for me.
Even though I made it work properly for a lot of images, I'm getting a 'math domain error' in the raw2temp module in some cases. Do you know why is this happening? All metadata that raw2temp needs are available so I guess that this shouldn't be happening.
Here you have some examples of the 'troublemakers' :)
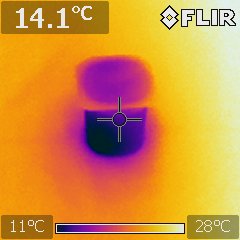
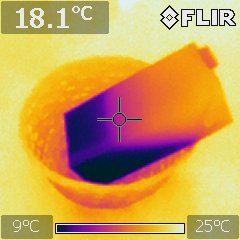
Thanks in advance. @Nervengift
The text was updated successfully, but these errors were encountered: