|
| 1 | +# 2125. Number of Laser Beams in a Bank |
| 2 | + |
| 3 | +- Difficulty: Medium. |
| 4 | +- Related Topics: Array, Math, String, Matrix. |
| 5 | +- Similar Questions: Set Matrix Zeroes. |
| 6 | + |
| 7 | +## Problem |
| 8 | + |
| 9 | +Anti-theft security devices are activated inside a bank. You are given a **0-indexed** binary string array `bank` representing the floor plan of the bank, which is an `m x n` 2D matrix. `bank[i]` represents the `ith` row, consisting of `'0'`s and `'1'`s. `'0'` means the cell is empty, while`'1'` means the cell has a security device. |
| 10 | + |
| 11 | +There is **one** laser beam between any **two** security devices **if both** conditions are met: |
| 12 | + |
| 13 | + |
| 14 | + |
| 15 | +- The two devices are located on two **different rows**: `r1` and `r2`, where `r1 < r2`. |
| 16 | + |
| 17 | +- For **each** row `i` where `r1 < i < r2`, there are **no security devices** in the `ith` row. |
| 18 | + |
| 19 | + |
| 20 | +Laser beams are independent, i.e., one beam does not interfere nor join with another. |
| 21 | + |
| 22 | +Return **the total number of laser beams in the bank**. |
| 23 | + |
| 24 | + |
| 25 | +Example 1: |
| 26 | + |
| 27 | +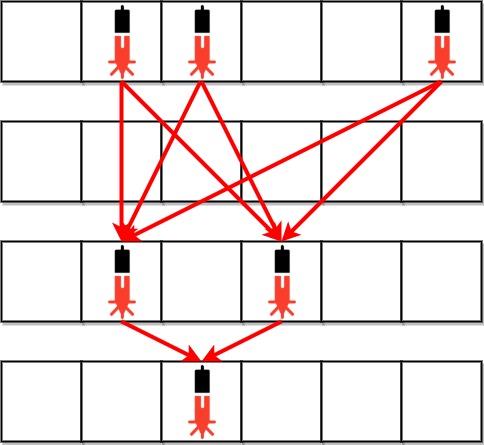 |
| 28 | + |
| 29 | +``` |
| 30 | +Input: bank = ["011001","000000","010100","001000"] |
| 31 | +Output: 8 |
| 32 | +Explanation: Between each of the following device pairs, there is one beam. In total, there are 8 beams: |
| 33 | + * bank[0][1] -- bank[2][1] |
| 34 | + * bank[0][1] -- bank[2][3] |
| 35 | + * bank[0][2] -- bank[2][1] |
| 36 | + * bank[0][2] -- bank[2][3] |
| 37 | + * bank[0][5] -- bank[2][1] |
| 38 | + * bank[0][5] -- bank[2][3] |
| 39 | + * bank[2][1] -- bank[3][2] |
| 40 | + * bank[2][3] -- bank[3][2] |
| 41 | +Note that there is no beam between any device on the 0th row with any on the 3rd row. |
| 42 | +This is because the 2nd row contains security devices, which breaks the second condition. |
| 43 | +``` |
| 44 | + |
| 45 | +Example 2: |
| 46 | + |
| 47 | +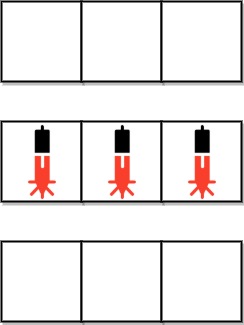 |
| 48 | + |
| 49 | +``` |
| 50 | +Input: bank = ["000","111","000"] |
| 51 | +Output: 0 |
| 52 | +Explanation: There does not exist two devices located on two different rows. |
| 53 | +``` |
| 54 | + |
| 55 | + |
| 56 | +**Constraints:** |
| 57 | + |
| 58 | + |
| 59 | + |
| 60 | +- `m == bank.length` |
| 61 | + |
| 62 | +- `n == bank[i].length` |
| 63 | + |
| 64 | +- `1 <= m, n <= 500` |
| 65 | + |
| 66 | +- `bank[i][j]` is either `'0'` or `'1'`. |
| 67 | + |
| 68 | + |
| 69 | + |
| 70 | +## Solution |
| 71 | + |
| 72 | +```javascript |
| 73 | +/** |
| 74 | + * @param {string[]} bank |
| 75 | + * @return {number} |
| 76 | + */ |
| 77 | +var numberOfBeams = function(bank) { |
| 78 | + var res = 0; |
| 79 | + var lastRowDeviceNum = 0; |
| 80 | + for (var i = 0; i < bank.length; i++) { |
| 81 | + var deviceNum = 0; |
| 82 | + for (var j = 0; j < bank[i].length; j++) { |
| 83 | + if (bank[i][j] === '1') deviceNum++; |
| 84 | + } |
| 85 | + if (deviceNum === 0) continue; |
| 86 | + res += lastRowDeviceNum * deviceNum; |
| 87 | + lastRowDeviceNum = deviceNum; |
| 88 | + } |
| 89 | + return res; |
| 90 | +}; |
| 91 | +``` |
| 92 | + |
| 93 | +**Explain:** |
| 94 | + |
| 95 | +nope. |
| 96 | + |
| 97 | +**Complexity:** |
| 98 | + |
| 99 | +* Time complexity : O(n * m). |
| 100 | +* Space complexity : O(1). |
0 commit comments